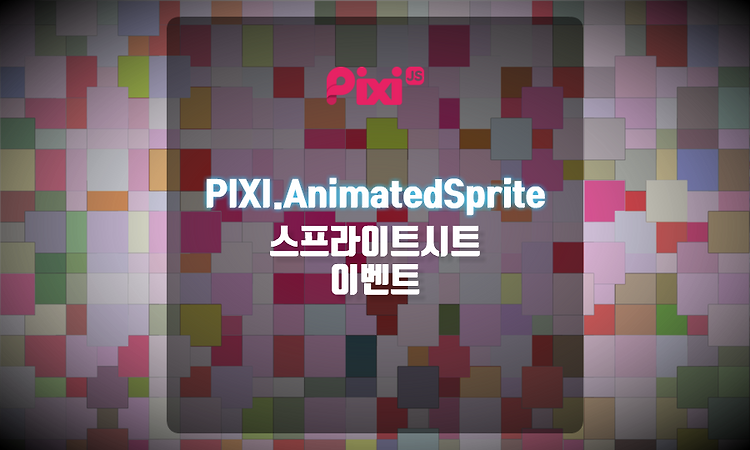
PIXI.AnimatedSprite #2 애니메이션 이벤트
천둥상어
·2024. 3. 2. 23:32
AnimatedSprite로 생성된 인스턴스는 단순히 재생만 가능한게 아니다.
Pixi.js의 AnimatedSprite는 몇가지 이벤트를 제공한다.
- onComplete
애니메이션의 프레임이 종료시 - onLoop
애니메이션이 프레임이 종료되고 다시 처음부터 시작시 - onFrameChange
프레임 변경시
애니메이션 이벤트 만들기
아래 코드는 캐릭터가 달리다가 클릭을 하면 점프를 하는 단순한 로직이다.
여기서는 onComplete를 이벤트를 이용해 점프시 점프 동작이 모두 끝나야
다시 달리기 상태로 돌아오게 하고 있다.
달리는 프레임
점프하는 프레임
코드 설명
위 두가지 상태의 이미지들을 불러와서 두개의 AnimatedSprite를 만들었다.
두 인스턴스는 동일한 컨테이너에 배치하였는데
달리는 인스턴스의 visble 값을 true,
점프하는 인스턴스의 visble 값은 fasle이다.
즉, 달리는 모습이 기본 IDLE 상태이며
점프는 캐릭터를 클릭시에만 재생이 되어야 한다.
캐릭터를 클릭하면 달리는 인스턴스의 visible 값을 false 처리 하고
점프하는 인스턴의 visible 값을 true처리 하여 보여준다.
점프하는 인스턴스는 onComplete 이벤트가 물려있다.
프레임이 끝까지 재생되면 콜백 함수를 호출하고
점스하는 인스턴스는 감추고 달리는 인스턴스를 다시 보여주게 된다.
참고로 변수 bJumping은 점프가 끝나는 동안 클릭에 의한 재호출을 방지하는 플래그 변수다.
import * as PIXI from 'pixi.js';
/**
* onComplete 애니메이션 종료시
* onLoop loop가 true인 경우 한번 돌때마다.
* onFrameChange 애니메이션 프레임 변경시
*/
export default class Exam_2 extends PIXI.Container {
constructor() {
super();
this.ct = new PIXI.Container();
this.addChild(this.ct);
this.ct.eventMode = 'static';
this.ct.cursor = 'pointer';
this.ct.on('pointertap', this.onTab, this);
this.runAnim;
this.jumpAnim;
this.bJumping = false;
this.init();
}
async init() {
for (let i = 0; i < 15; i++) {
await PIXI.Assets.load([`flatboy/run/run_${i}.png`]);
await PIXI.Assets.load([`flatboy/jump/jump_${i}.png`]);
}
const run_textures = [];
const jump_textures = [];
for (let j = 0; j < 15; j++) {
run_textures.push(PIXI.Assets.cache.get(`flatboy/run/run_${j}.png`));
jump_textures.push(PIXI.Assets.cache.get(`flatboy/jump/jump_${j}.png`));
}
this.runAnim = new PIXI.AnimatedSprite(run_textures);
this.runAnim.onLoop = () => this.onRunLoop();
this.runAnim.scale.set(0.2, 0.2);
this.runAnim.animationSpeed = 1 / 2; // 2 fps
this.runAnim.loop = true;
this.runAnim.play();
this.ct.addChild(this.runAnim);
this.jumpAnim = new PIXI.AnimatedSprite(jump_textures);
this.jumpAnim.onComplete = () => this.onJumpComplete();
this.jumpAnim.onFrameChange = ($currentFrame) =>
this.onJumpFrameChange($currentFrame);
this.jumpAnim.scale.set(0.2, 0.2);
this.jumpAnim.animationSpeed = 1 / 2; // 2 fps
this.jumpAnim.loop = false;
this.jumpAnim.visible = false;
this.ct.addChild(this.jumpAnim);
this.ct.position.set(160, 200);
}
onTab($evt) {
if (this.bJumping) return;
this.bJumping = true;
this.runAnim.visible = false;
this.runAnim.stop();
this.jumpAnim.gotoAndPlay(0);
this.jumpAnim.visible = true;
}
onRunLoop() {
console.log('loop');
}
onJumpComplete() {
this.jumpAnim.visible = false;
this.jumpAnim.stop();
this.runAnim.gotoAndPlay(1);
this.runAnim.visible = true;
this.bJumping = false;
}
onJumpFrameChange($currentFrame) {
//console.log($currentFrame);
}
}
실행 결과
'프로그래밍 > Pixi.js7' 카테고리의 다른 글
pixi-sound 사용하기 (0) | 2024.03.27 |
---|---|
PIXI.Graphics GPU 최적화 (0) | 2024.03.19 |
PIXI.AnimatedSprite #1 애니메이션 재생 (0) | 2024.02.18 |
PIXI.Assets 로드된 이미지에서 특정 영역만 렌더링하기 (1) | 2024.02.14 |
PIXI.Assets 리소스 불러오기 (4) | 2024.02.05 |